A Stock Market API in 30 Minutes
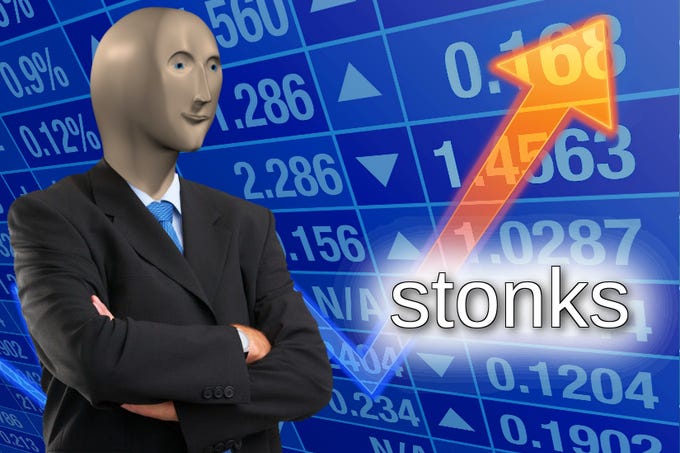
Since everyone in my entire life is buying and selling GameStop this month, a friend and I started making a small app to visualize stock market data and chat with your friends. He wanted to practice Tailwind and Svelte, I wanted to use Go and GRPC....
Well, I didn't end up using Go.
I was researching the best api's to get stock market data, and realized e…
Keep reading with a 7-day free trial
Subscribe to zach.codes to keep reading this post and get 7 days of free access to the full post archives.