Nexus + Prisma: the Future of Backend
Setup GraphQL with Nexus + Prisma. Automate code generation, TypeScript generation, and crud resolvers.
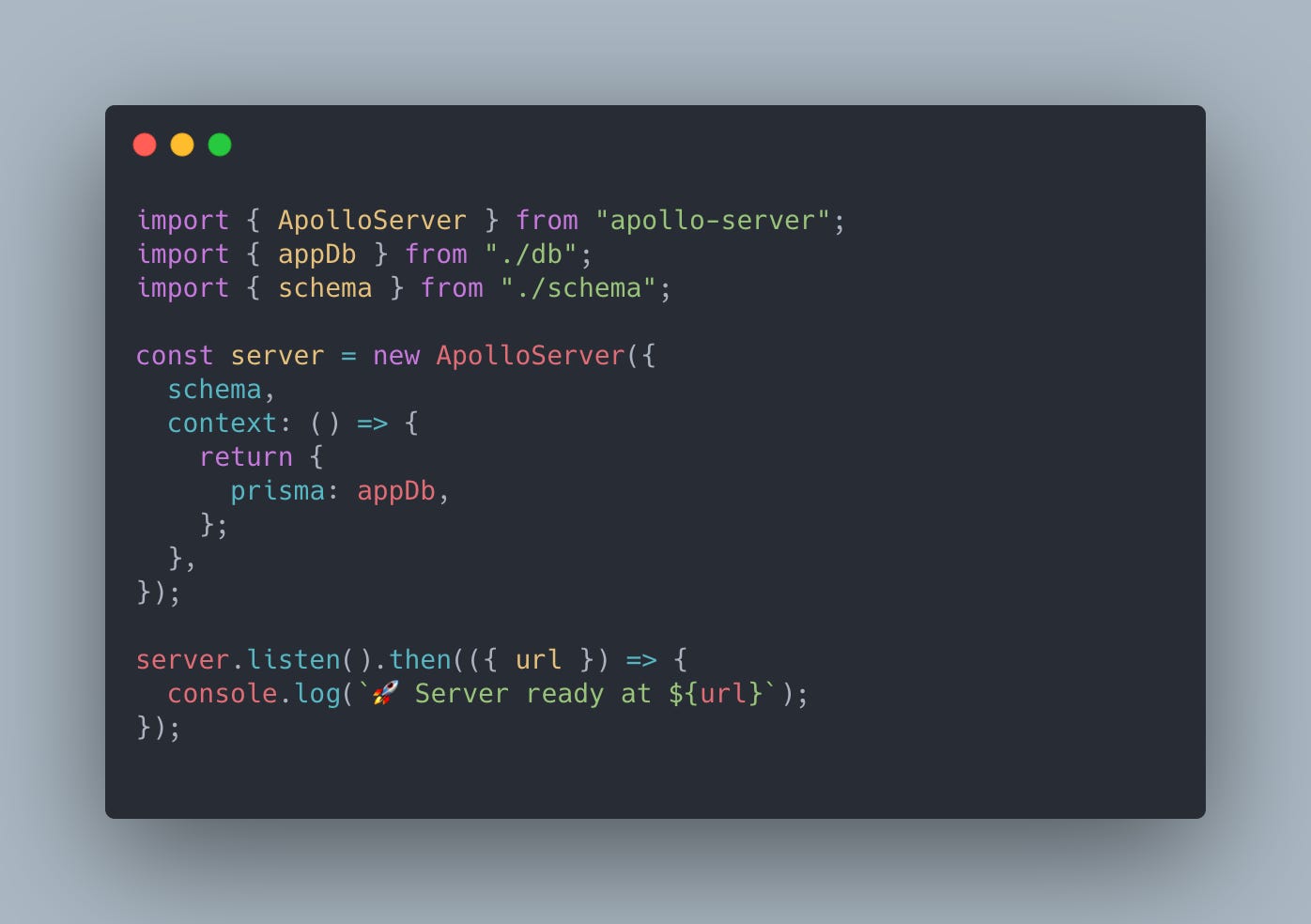
GraphQL Nexus and Prisma is the future of backend development. It has the instant feedback you'd expect in 2021, and automates resolvers for common operations.
You can view the source code for this post on github
It does all of this while staying our of our way. Letting you build fully custom logic, while taking advantage of automated features for the sim…
Keep reading with a 7-day free trial
Subscribe to zach.codes to keep reading this post and get 7 days of free access to the full post archives.