Building a GraphQL Client For File Uploads
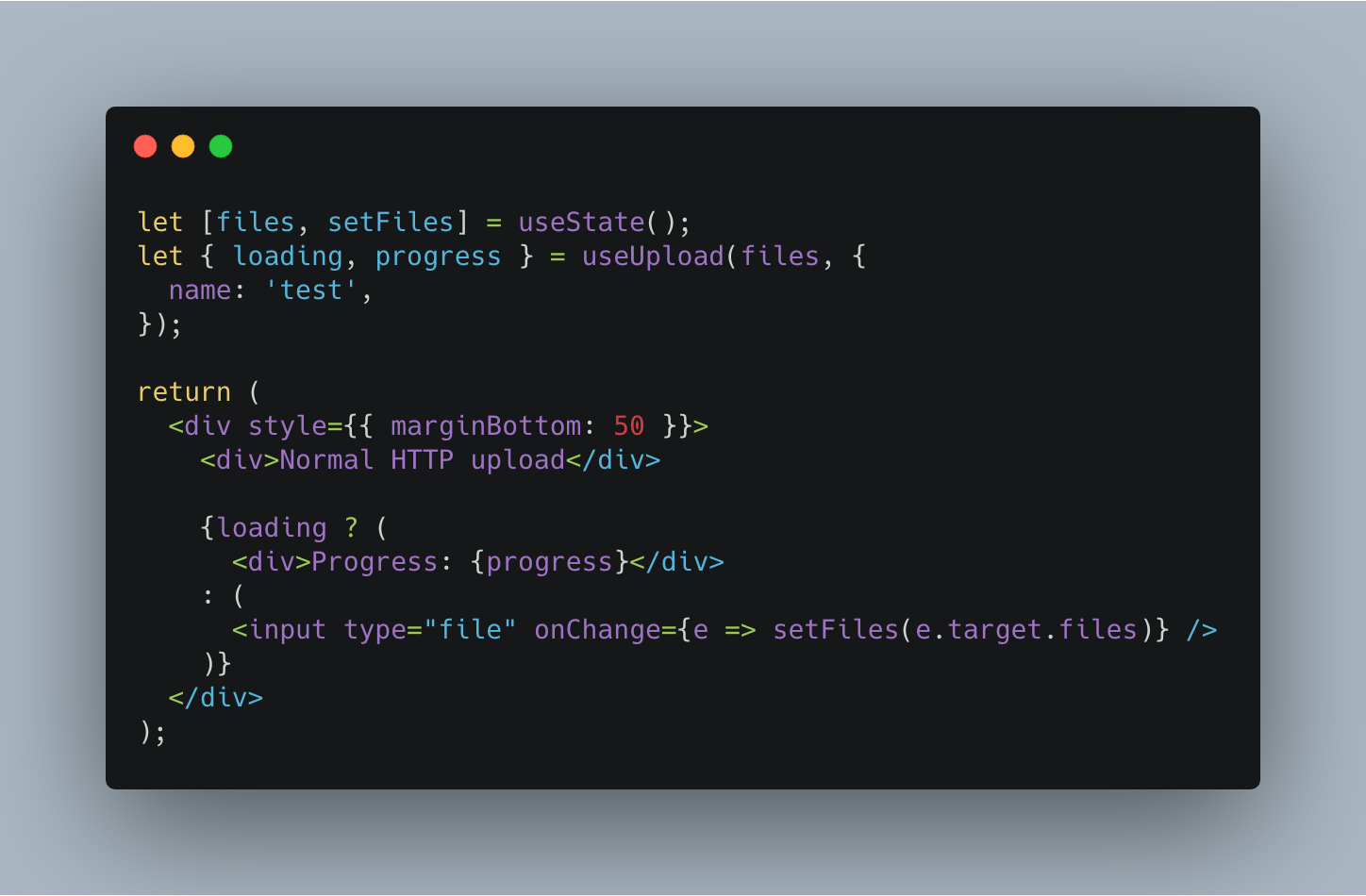
Doing normal GraphQL requests is straight forward, as documented on graphql.org. Anyone can use a normal fetch
or curl
to do basic requests. However, I haven't seen anyone doing what I am working on. File upload progress.
Since the spec is only in Apollo Server, and not the main GraphQL spec, it seems even harder to figure out how it should be done. (Please correct me if this is wrong). In order to get file progress in JS, you still must use XHR. All the current clients use fetch, which doesn't support this, and most do not have built in file uploading. In this post, I am going to detail my process for building react-use-upload, and how I made my code conform to the spec.
Getting started
When I set out building this library, I wanted to get file uploads working with a normal REST api. This helped me lay a solid foundation for my library. After, I first took a look at how graphql-tag works:
import gql from 'graphql-tag';
const uploadMutation = gql`
mutation UploadFile($input: UploadFile…
Keep reading with a 7-day free trial
Subscribe to zach.codes to keep reading this post and get 7 days of free access to the full post archives.